Chapter 6 VICAL in GEE
This section shows how to use the VICAL scripts to implement them in GEE.
VICAL has three main files that can be imported into a GEE Script, these are:
// Image collections
var imp = require('users/InifapCenidRaspa/VICAL:Exportaciones');
// Vegetation indices
var imp2= require('users/InifapCenidRaspa/VICAL:VegetationIndex');
// visualization styles
var St= require('users/InifapCenidRaspa/VICAL:Style');
6.1 Image collection
Before importing the image collections, some variables must be declared that are useful for filtering this collection: i) a point or polygon; ii) date range, and iii) cloud threshold value in images. These declarations are shown in the following code:
var fecha = ['2021-01-01', '2022-03-18']; //Start and end date
//polygon or point
var table = ee.FeatureCollection("projects/calcium-verbena-328905/assets/Bate");
var p_nubes= 30;//percentage of clouds
6.1.1 Landsat
If you want to use cloud-free atmospherically corrected LandSat images (4, 5, 7, 8 and 9), you can use the following code. A function is created to join the image collections. To do this, use the imp file.
function ColeccionImagenSR(fecha, recorte, umbral)
{// image collections are imported using the "imp" file
var L9sr = imp.ColeccionLandsatSR(fecha, 'LC09', recorte, umbral);
var L8sr = imp.ColeccionLandsatSR(fecha, 'LC08', recorte, umbral);
var L7sr = imp.ColeccionLandsatSR(fecha, 'LE07', recorte, umbral);
var L5sr = imp.ColeccionLandsatSR(fecha, 'LT05', recorte, umbral);
var L4sr = imp.ColeccionLandsatSR(fecha, 'LT04', recorte, umbral);
//ETM and ETM+ data are spectral fit to OLI and OLI-2
var L7a = L7sr.map(imp.TMaOLI);
var L5a = L5sr.map(imp.TMaOLI);
var L4a = L4sr.map(imp.TMaOLI);
// Join collections
var serieT =L9sr.merge(L8sr).merge(L7a).merge(L5a).merge(L4a).sort('system:time_start');
return serieT;
}//The collection is imported using the previous function
var l8Sergio=ColeccionImagenSR(fecha, table, p_nubes);
//we can print the images using the print() function to see if the
//filtering of the image collection has been carried out (Figure 6.1)
print (l8Sergio);
With these image collections, time series of different vegetation indices can be calculated.
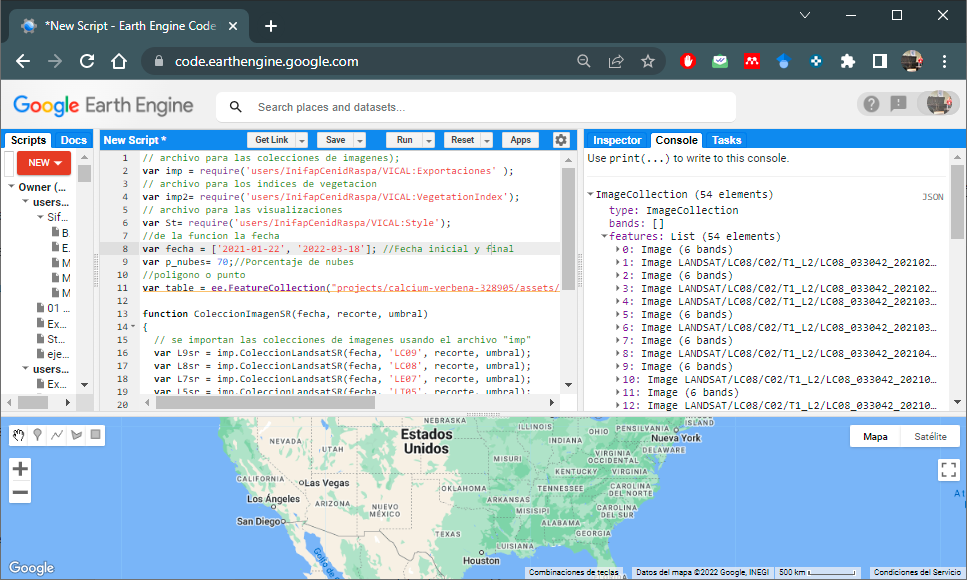
Figure 6.1: Landsat Image Collection
6.1.2 Sentinel-2
If you want to use cloud-free, atmospherically corrected Sentinel-2 images, you can use the following code.
//The collection of images is imported using the following code
var S2sr = imp.ColeccionImagenSentinelSR(fecha, table, p_nubes);
//we can print the images using the print() function to see if the
//filtering of the image collection has been carried out (Figure 6.2)
print (S2sr);
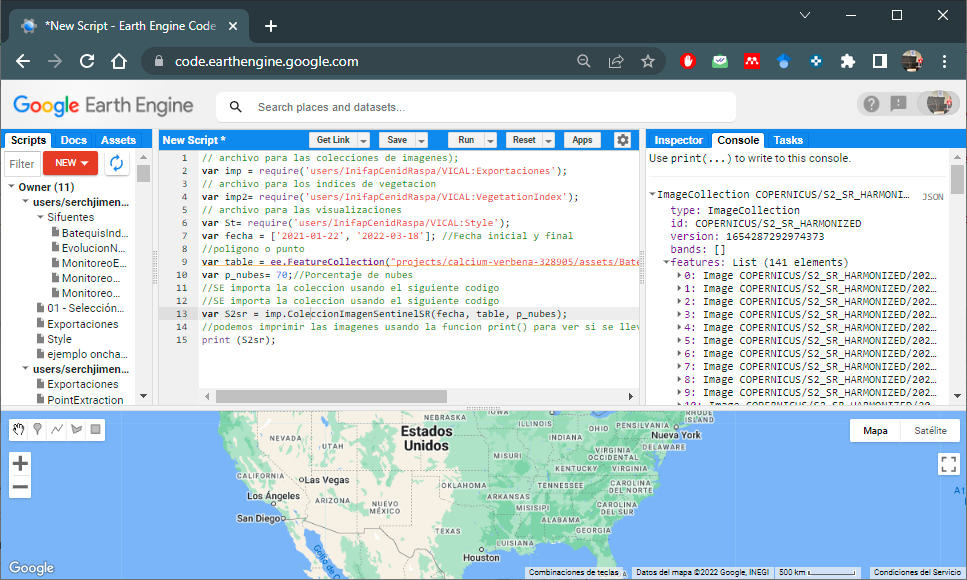
Figure 6.2: Sentinel-2 Image Collection
6.1.3 Landsat y Sentinel-2
If you want to use cloud-free, atmospherically corrected LandSat and Sentinel-2 images, you can use the following code, data were spectrally fit to Landsat 8 bands. The functions described in Section 6.1:
function ColeccionImagenAMBOS(fecha, recorte, umbral)
{//Function for Landsat images with spectral adjustment
var L8Conjunto=ColeccionImagenSR(fecha, recorte, umbral)
//Sentinel
var S2sr = imp.ColeccionImagenSentinelSR(fecha, recorte, umbral);
//Spectral matching of sentinel-2 to Landsat
var S2a = S2sr.map(imp.MSIaOLI);
var serieT = S2a.merge(L8Conjunto).sort('system:time_start');
return serieT;
}
//The collection is imported
var S2B = ColeccionImagenAMBOS(fecha, table, p_nubes);
//we can print the images using the print() function to see if the
//filtering of the image collection has been carried out (Figure 6.3)
print (S2sr);
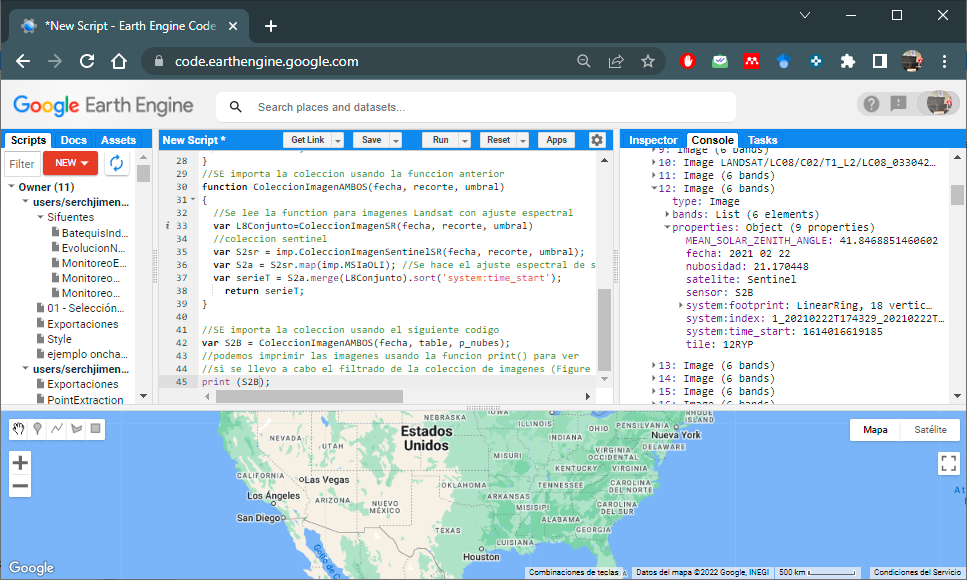
Figure 6.3: Landsat and Sentinel-2 imagery collection
To view an example script click here
6.2 Vegetation indices
To calculate some of the VIs of VICAL you have to use the file imp2; and these VIs are imported using the names of the ExpresionGEE column that are shown in the Table 6.1.
For example, to calculate NDVI with LandSat and Sentinel-2 images from section 6.1.3, the following code would be used:
//Normalized Difference Vegetation Index- NDVI
var ivs = ee.ImageCollection(S2B.map(imp2.NDVI));
//Print and view the NDVI band
print (ivs);
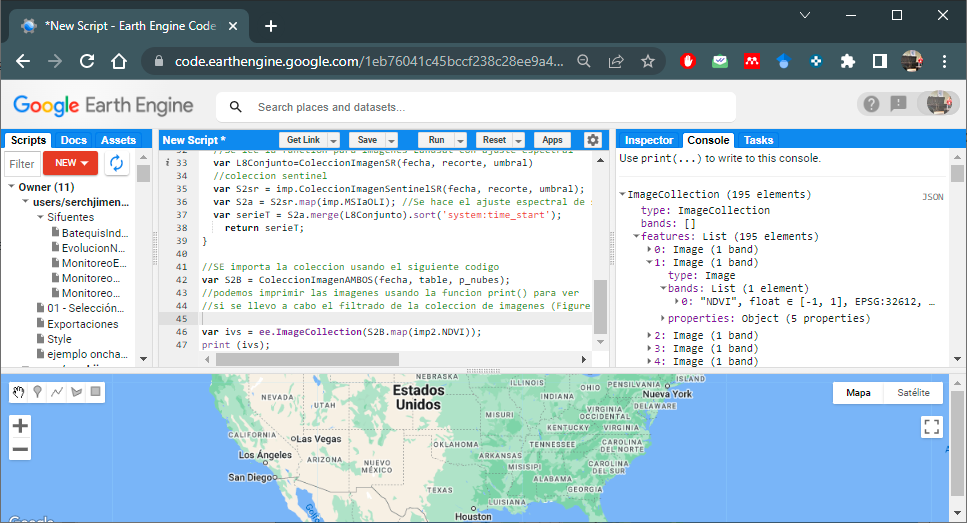
Figure 6.4: NDVI Band Image Collection
The following code shows an example to display on the map the NDVI of the first image of the collection and cropped for the area. The st file of ¨VICAL is used.
//NDVI from the first image in the collection
var iv = ivs.first();
//Color palette where 'st' file is used
var ivVis = {min :0, max : 1, palette : St.paletaIV};
Map.addLayer(iv.clip(table), ivVis,'NDVI'); //Indice
//the map is centered to the area
Map.centerObject(table, 13);
Figure 6.5 shows the NDVI map for the area of interest
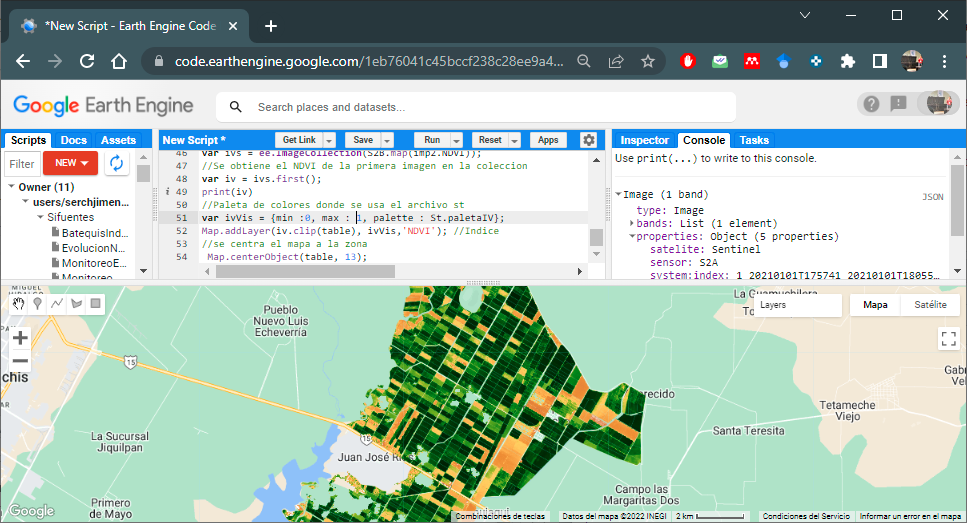
Figure 6.5: NDVI map for the area of interest
To view the sample code click here
If you want to display the NDVI of a particular image, you must convert it to a list.
Number | Index | Abbreviation | ExpresionGEE | Coefficients |
---|---|---|---|---|
1 | Atmospherically resistant vegetation index | ARVI* | ARVI | γ=1.0 |
2 | Adjusted transformed soil-adjusted vegetation index | ATSAVI* | ATSAVI | |
3 | Difference vegetation index | DVI | DVI | |
4 | Enhanced vegetation index | EVI | EVI | C1=6.0, C2= 7.5; L=1.0 |
5 | Enhanced vegetation index | EVI2* | EVI2 | C1=2.4 |
6 | Green normalized difference vegetation index | GNDVI | GNDVI | |
7 | Modified soil adjusted vegetation index | MSAVI2 | MSAVI2 | |
8 | Moisture stress index | MSI | MSI | |
9 | Modified triangular vegetation index | MTVI | MTVI | |
10 | Modified triangular vegetation index-2 | MTVI2 | MTVI2 | |
11 | Normalized difference tillage index (NDTI) | NDTI | NDTI | |
12 | Normalized difference vegetation index | NDVI | NDVI | |
13 | Normalized difference water index | NDWI | NDWI | |
14 | Optimized soil adjusted vegetation index | OSAVI* | OSAVI | X=0.16 |
15 | Renormalized difference vegetation index | RDVI | RDVI | |
16 | Redness index | RI | RI | |
17 | Ratio vegetation index | RVI | RVI | |
18 | Soil adjusted vegetation index | SAVI* | SAVI | L=0.5 |
19 | Triangular vegetation index | TVI | TVI | |
20 | Transformed soil adjusted vegetation index | TSAVI* | TSAVI | a= 1 ; b=0; |
21 | Visible atmospherically resistant index | VARI | VARI | |
22 | Vegetation index number or simple ratio | VIN | VIN | |
23 | Wide dynamic range vegetation index | WDRVI* | WDRVI | α=0.2 |
6.3 GithUb repository
VICAL codes are written in JavaScript and are freely available on GitHub (https://www.github.com/CenidRaspaRiego/VICAL (accessed on 16 June 2022))